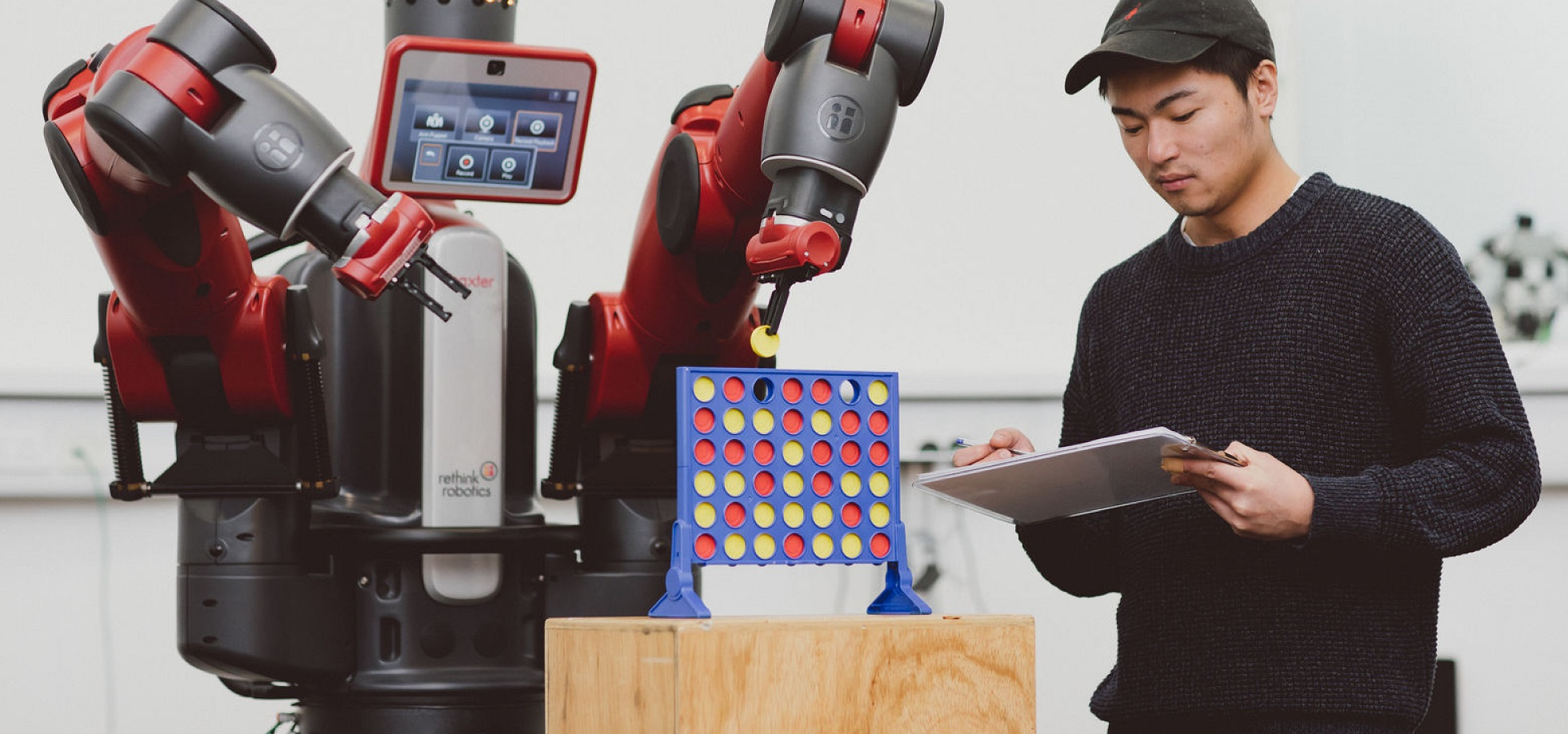
Aims
Data structures and algorithms lie at the heart of Computer Science as they are the basis for the efficient solution of programming tasks. In this module, students will study core algorithms and data structures, as well as being given an introduction to algorithm analysis and basic computability.
The module will give students core algorithmic skills that are required for Years 2 and 3 of the Computer Science degree schemes.
Learning Outcomes
After completing this module, students will be expected to be able to:
1. Demonstrate an understanding of core data types such as stacks, queues, trees, and graphs.
2. Implement core data types in Java and write programs that make efficient use of them.
3. Reason about the time and space complexity of programs.
4. Demonstrate knowledge of commonly used algorithms.
5. Explain the main concepts of computability and how some problems have no algorithmic solution.
Syllabus
Data types
Abstract data types
Lists, stacks, queues, trees, sets, graphs
Algorithms
Divide and conquer
Sorting and searching
Algorithms: binary search trees, minimum cost spanning trees, shortest paths, parse trees
Algorithm analysis: time and space complexity
Basic computability, incomputable functions and the halting problem
- Module Supervisor: David Richerby